Introduction
Error When Opening a Sketch in Arduino IDE can occur due to several reasons, such as a corrupted file, incorrect file path, or incompatible Arduino IDE version. If the sketch file is damaged or incomplete, the IDE may fail to open it properly. Additionally, if the file is not inside a correctly named folder matching the sketch name, the Arduino IDE may not recognize it. Permission issues, missing libraries, or workspace errors can also cause this problem. To fix it, ensure the file structure is correct, check for missing dependencies, and try reopening the sketch in a newly installed Arduino IDE version.
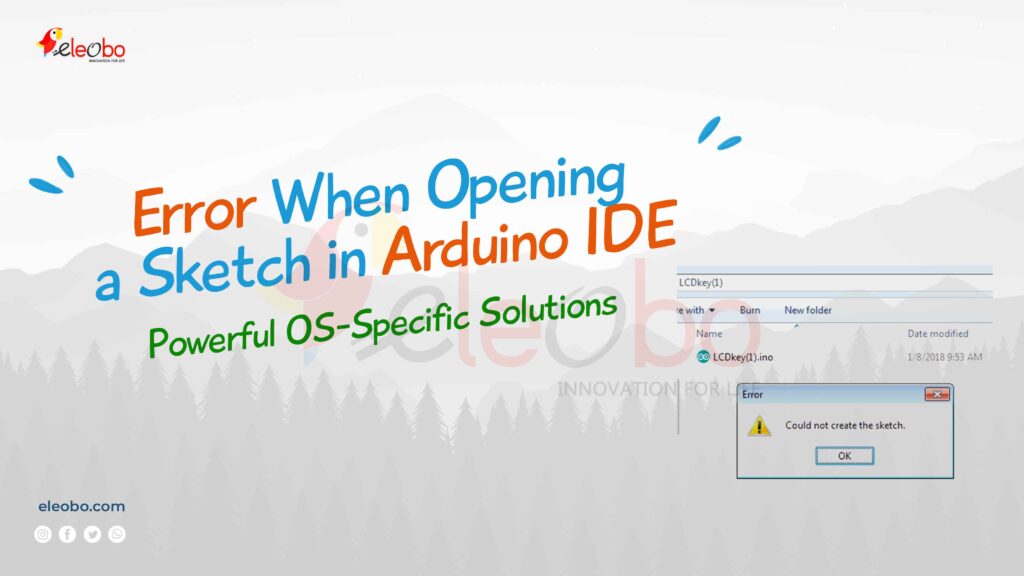
Table of Contents
Windows-Specific Errors and Solutions
Error 1: “Access Denied” in Windows
Error Message: "Error when opening a sketch in Arduino IDE: Access is denied"
Quick Fix:
- Right-click Arduino IDE
- Select “Run as administrator”
- Try opening the sketch again
Permanent Fix:
- Find Arduino IDE shortcut
- Right-click → Properties
- Compatibility tab
- Check “Run this program as administrator”
- Apply → OK
Error 2: “File Path Too Long” in Windows
Error Message: "Cannot open sketch in deeply nested folders"
Solution:
Move the sketch to a shorter path:
Bad: C:\Users\Name\Documents\Arduino\Projects\2024\LED\Version2\sketch.ino
Good: C:\Arduino\Projects\LED\sketch.ino
Error 3: Windows Antivirus Blocking
Error Message: "Error when opening a sketch in Arduino IDE: File is in use by another program"
Fix:
- Open Windows Security
- Virus & threat protection → Manage settings
- Add exclusion → Folder
- Select the Arduino sketch folder
Linux-Specific Errors and Solutions
Error 1: Permission Denied in Linux
Error Message: "Error when opening a sketch in Arduino IDE: Permission denied"
Terminal Fix:
ls -l sketch.ino # Check file ownership
chmod 644 sketch.ino # Fix permissions
sudo chown $USER:$USER sketch.ino # Change ownership if needed
Error 2: Locked File in Linux
Error Message: "File is locked or in use"
Solution:
lsof | grep sketch.ino # Find processes using the file
kill -9 [PID] # Kill the blocking process
Error 3: Hidden Files Issue
Error Message: "Cannot find sketch"
Fix:
ls -la ~/Arduino # Show hidden files
chmod -R 755 ~/.arduino # Fix hidden file permissions
Mac-Specific Errors and Solutions
Error 1: macOS Security Block
Error Message: "Arduino IDE cannot open sketch due to security settings"
Solution:
- System Preferences → Security & Privacy
- Click the lock to make changes
- Allow Arduino IDE
- Restart Arduino IDE
Error 2: Mac File Quarantine
Error Message: "File is damaged and cannot be opened"
Terminal Fix:
xattr -d com.apple.quarantine /Applications/Arduino.app # Remove quarantine attribute for Arduino IDE
xattr -d com.apple.quarantine ~/Documents/Arduino/sketch.ino # For sketch files
Error 3: Mac File Lock
Error Message: "File is locked"
Fix:
- Select the sketch file
- Press Cmd + I (Get Info)
- Uncheck “Locked”
- Try opening it again
Universal Solutions (All Operating Systems)
Error 1: Corrupted Sketch File
The error “Corrupted Sketch File” in Arduino usually means that the .ino file you are trying to open is damaged, incomplete, or unreadable. Here are some possible reasons and solutions:
Possible Causes & Fixes
File Not Fully Saved or Interrupted
- If the Arduino IDE crashed while saving, the file might be incomplete.
- Fix: Try opening the file with a text editor (Notepad++, VS Code) to check if the content is intact.
File Encoding Issues
- If the sketch file is saved in a different encoding, the Arduino IDE might not recognize it.
- Fix: Open it in a text editor and resave it using UTF-8 encoding.
Corrupted Data Due to Power Failure or System Crash
- If your system shut down unexpectedly while editing the sketch, the file may be corrupted.
- Fix: Check if you have an autosaved backup in the Arduino temporary folder (
C:\Users\YourUser\AppData\Local\Temp\
on Windows).
Incorrect File Format
- If the file was renamed incorrectly or saved in another format (e.g.,
.txt
instead of.ino
), the IDE won’t recognize it. - Fix: Ensure the file has a
.ino
extension.
File Opened in Another Program
- If the file is open in another application (e.g., Word, Excel), it might be locked.
- Fix: Close all other programs that might be using the file and try opening it again.
Disk Errors or Bad Sectors
- If the file is on a corrupted storage device, it may not open properly.
- Fix: Move the file to a different location and try opening it.
How to Recover a Corrupted Sketch?
- Check Backups: If you use GitHub or cloud storage (Google Drive, OneDrive), try restoring an older version.
- Use Arduino Autosave: Check the temporary folder for any auto-saved versions.
- Recreate the File: If nothing works, create a new
.ino
file and manually copy-paste your code from the corrupted file if possible.
Symptoms:
- Strange characters in code
- Missing portions
- File won't open
Recovery Steps:
- Create a new sketch:
void setup() {
Serial.begin(9600);
}
void loop() {}
- Copy a working backup
- Save with a new name
Error 2: IDE Cache Problems
IDE Cache Problems in Arduino: What It Means & How to Fix It
The “IDE Cache Problems” in the Arduino IDE usually occur due to temporary files that store previous settings, compilation results, or dependencies. When these files become outdated or corrupted, they can cause issues like:
🔹 Compilation errors
🔹 Sketch not uploading properly
🔹 Libraries not updating correctly
🔹 Arduino IDE behaving unexpectedly
🛠️ How to Fix Arduino IDE Cache Problems
✅ Method 1: Clear the Cache Manually
- Close the Arduino IDE before making any changes.
- Navigate to the cache folder based on your OS:
- Windows:
C:\Users\YourUsername\AppData\Local\Arduino15\cache\
- Mac:
~/Library/Arduino15/cache/
- Linux:
~/.arduino15/cache/
- Windows:
- Delete the contents of the cache folder.
- Restart the Arduino IDE and try again.
✅ Method 2: Clear Temporary Compilation Files
- Go to Arduino Preferences
- Open File → Preferences (or Ctrl + , shortcut).
- Check the “Show verbose output during compilation” option.
- Find the Build Folder
- The output window will show the temporary directory where compiled files are stored.
- It usually looks like:
C:\Users\YourUsername\AppData\Local\Temp\arduino_build_xxxxxx
- Delete the Arduino Build Folder
- Close the IDE.
- Navigate to the above location and delete all folders inside
arduino_build_xxxxxx
.
✅ Method 3: Reinstall the Arduino IDE
If clearing the cache does not fix the problem, try reinstalling the Arduino IDE:
- Uninstall Arduino from your system.
- Delete the Arduino15 Folder
- Windows:
C:\Users\YourUsername\AppData\Local\Arduino15\
- Mac:
~/Library/Arduino15/
- Linux:
~/.arduino15/
- Windows:
- Reinstall the Latest Arduino IDE from Arduino’s official website.
🚀 Prevention Tips
✔ Regularly clear cache if you frequently update libraries and boards.
✔ Avoid sudden shutdowns while the IDE is compiling.
✔ Use Arduino IDE Portable Mode to prevent system-wide cache issues.
If you still have issues, let me know the exact error message, and I’ll help further! 😊
Symptom: Multiple sketches won't open
Clear Cache:
Windows:
del %APPDATA%\Arduino15\cache
Linux:
rm -rf ~/.arduino15/cache
Mac:
rm -rf ~/Library/Arduino15/cache
Error 3: Workspace Issues in Arduino – What It Means & How to Fix It
The “Workspace Issues” error in the Arduino IDE usually refers to problems related to:
🔹 Sketch location (workspace directory issues)
🔹 Incorrect file paths
🔹 Permission issues
🔹 Corrupt or missing files in the project folder
🛠️ How to Fix Workspace Issues in Arduino
1. Check Sketch Location
- Make sure your
.ino
file is inside a properly named sketch folder.- Example: If your file is
my_project.ino
, it must be inside a folder namedmy_project/
. - If it’s outside or in a different directory, move it inside a correctly named folder.
- Example: If your file is
2. Avoid Special Characters in File Path
- Ensure the project folder does not have spaces, special characters, or non-English characters.
- ❌
C:\Arduino Projects\My#Sketch!.ino
- ✅
C:\Arduino_Projects\MySketch\MySketch.ino
- ❌
3. Run Arduino IDE as Administrator (Windows)
- Sometimes, Arduino does not have the right permissions to access files.
- Fix: Right-click the Arduino IDE icon → Run as Administrator.
4. Check Read/Write Permissions (Mac/Linux)
- If using Mac or Linux, ensure the workspace has the correct permissions:
sudo chmod -R 777 ~/Arduino
- This will give full access to the Arduino folder.
5. Reset the Arduino IDE Workspace
- Go to File → Preferences and note down the Sketchbook location.
- Change it to a simpler path like
C:\Arduino_Projects\
(Windows) or~/Arduino/
(Mac/Linux). - Restart the IDE and check if the issue is resolved.
6. Reinstall the Arduino IDE
If none of the above steps work, try uninstalling and reinstalling the Arduino IDE:
- Uninstall Arduino from your system.
- Delete the Arduino15 Folder (to remove corrupted settings):
- Windows:
C:\Users\YourUsername\AppData\Local\Arduino15\
- Mac:
~/Library/Arduino15/
- Linux:
~/.arduino15/
- Windows:
- Reinstall the latest Arduino IDE from Arduino’s official website.
Final Tips
✔ Always keep your Arduino projects inside a simple directory like C:\Arduino_Projects\
.
✔ Avoid renaming, moving, or deleting files while the Arduino IDE is open.
✔ Check if your antivirus or security software is blocking file access.
If you’re still facing issues, let me know the exact error message, and I’ll help you troubleshoot further! 😊
Symptom: All sketches fail to open
Reset Workspace:
Windows:
- Delete %APPDATA%\Arduino15
- Restart IDE
Linux:
rm -rf ~/.arduino15
Mac:
rm -rf ~/Library/Arduino15
Prevention Steps (All OS)
1. Regular Backups
// Use version numbering
sketch_v1.ino
sketch_v2.ino
2. File Structure
Arduino/
|-- Projects/
|-- Current/
|-- Backups/
|-- Libraries/
3. Maintenance
Weekly:
1. Backup sketches
2. Clear cache
3. Update IDE
When to Reinstall Arduino IDE
Windows:
- Control Panel → Programs
- Uninstall Arduino
- Download a fresh copy
- Run the installer as admin
Linux:
sudo apt-get remove arduino
sudo apt-get purge arduino
sudo apt-get update
sudo apt-get install arduino
Mac:
- Delete Arduino from Applications
- Download a new version
- Drag to Applications
- First launch: Right-click → Open
Additional Resources
Official Support:
- Windows: Arduino Windows Guide
- Linux: Arduino Linux Guide
- Mac: Arduino macOS Guide
Community Help:
- Arduino Forums
- Stack Exchange
- GitHub Issues
- Local Maker Groups
Remember: When encountering an error when opening a sketch in Arduino IDE, try OS-specific solutions first, then universal fixes if needed. Stay proactive with regular maintenance to prevent these issues in the future!
Download BlueBot Controller App and start your journey today!