Have you ever struggled to create custom graphics for your microcontroller projects? If so, you’re not alone. Many makers and electronics enthusiasts face this challenge daily. Fortunately, an OLED icon generator can solve this problem effectively. This powerful tool enables you to design pixel-perfect graphics for those tiny but mighty OLED displays that have become increasingly popular in DIY electronics projects.
OLED Icon Generator
Create pixel art icons for OLED displays and generate Arduino code.
OLED Screen Preview
Example Icons
Table of Contents
In this comprehensive guide, we’ll explore everything you need to know about using an OLED icon generator to elevate your projects. From basic principles to advanced techniques, you’ll learn how to create eye-catching icons that will make your devices stand out from the crowd. Moreover, we’ll introduce you to our custom-built web-based OLED icon generator that simplifies the entire process.
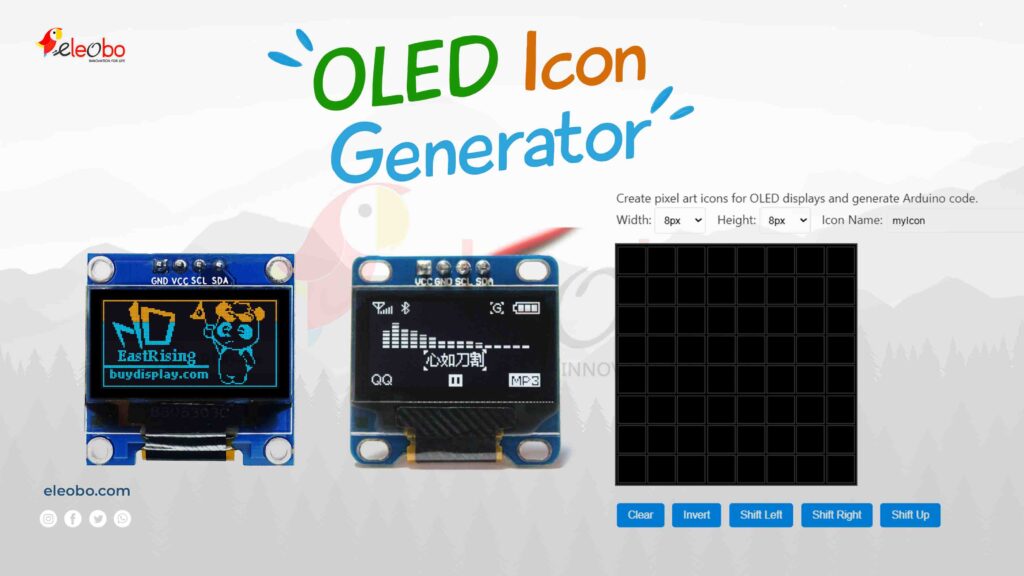
Sample Arduino code
Here’s a sample Arduino code that demonstrates how to use the icons generated by the OLED Icon Generator with an SSD1306 OLED display. This code includes one of the example icons (the “Heart”) and shows how to display it:
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
// OLED display dimensions
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
// Initialize OLED display with I2C address 0x3C
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
// Heart 8x8 icon from the example
const unsigned char heartIcon[] PROGMEM = {
0x66, // 01100110
0xff, // 11111111
0xff, // 11111111
0xff, // 11111111
0x7e, // 01111110
0x3c, // 00111100
0x18, // 00011000
0x00 // 00000000
};
void setup() {
// Initialize Serial for debugging
Serial.begin(115200);
// Initialize OLED display
if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;); // Don't proceed, loop forever
}
// Clear the display buffer
display.clearDisplay();
// Draw the heart icon at position (0,0)
display.drawBitmap(0, 0, heartIcon, 8, 8, WHITE);
// Display the buffer contents
display.display();
}
void loop() {
// Main loop can be empty if we're just showing a static icon
// Optional: Add animation by moving the icon
display.clearDisplay();
// Calculate position (example: bounce effect)
static int xPos = 0;
static int xDir = 1;
xPos += xDir;
if (xPos >= SCREEN_WIDTH - 8 || xPos <= 0) {
xDir = -xDir;
}
// Draw icon at new position
display.drawBitmap(xPos, 0, heartIcon, 8, 8, WHITE);
display.display();
delay(50); // Control animation speed
}
// Example function to display any generated icon
void displayIcon(const unsigned char* icon, int width, int height, int x, int y) {
display.clearDisplay();
display.drawBitmap(x, y, icon, width, height, WHITE);
display.display();
}
To use this code:
Required Libraries:
- Install “Adafruit_GFX” library
- Install “Adafruit_SSD1306” library
through the Arduino Library Manager
Hardware Setup:
- Connect your SSD1306 OLED display to your Arduino:
- SDA to Arduino SDA pin (A4 on Uno)
- SCL to Arduino SCL pin (A5 on Uno)
- VCC to 3.3V or 5V (depending on your display)
- GND to GND
How to Use Generated Icons:
- Copy the array generated from the OLED Icon Generator tool (e.g., the code in the textarea)
- Paste it into your Arduino sketch
- Use it with
display.drawBitmap()
as shown in the example
Here’s how to add and use a custom icon from the generator:
// Add your custom icon (example output from the generator)
const unsigned char myIcon[] PROGMEM = {
// Paste your generated bytes here
0x00, 0x00, 0x00, 0x00, // Replace with your icon data
// ... more bytes depending on size
};
void setup() {
// ... existing setup code ...
// Draw your custom icon
display.clearDisplay();
display.drawBitmap(0, 0, myIcon, 16, 16, WHITE); // Adjust width and height as needed
display.display();
}
Key features of this code:
- Uses PROGMEM to store the icon in flash memory
- Includes basic animation example
- Works with any icon size generated by the tool (just adjust width and height parameters)
- Compatible with common 128×64 OLED displays
Notes:
- Adjust the I2C address (0x3C) if your display uses a different address
- Modify the animation in loop() or remove it for static display
- Add multiple icons and switch between them as needed
- The display buffer is cleared before each draw to prevent overlapping
This code provides a starting point that you can modify based on your specific needs and the icons you create with the generator tool.
Why OLED Displays Are Revolutionary for Makers
Before diving into the specifics of using an OLED icon generator, let’s understand why OLED displays have become so popular in the maker community. Unlike traditional LCD screens, OLED displays offer superior contrast ratios, wider viewing angles, and remarkably low power consumption. Additionally, their self-illuminating pixels eliminate the need for backlighting, resulting in thinner, lighter displays perfect for portable projects.
Arduino enthusiasts, in particular, have embraced OLED displays for their projects because they provide a sleek, modern way to display information without consuming excessive power or requiring extensive wiring. Furthermore, these displays work seamlessly with popular microcontrollers like Arduino, ESP8266, and ESP32 through simple I2C or SPI interfaces.
The Challenge of Creating Custom Graphics
Despite their advantages, OLED displays present one significant challenge: creating custom graphics. Unlike text, which can be displayed using simple commands, graphics require pixel-by-pixel definitions usually stored as byte arrays in your code. Consequently, designing icons manually can be tedious and error-prone.
This is precisely where an OLED icon generator becomes invaluable. Rather than painstakingly calculating each byte value, you can visually design your images and automatically generate the corresponding code. As a result, you save countless hours and avoid frustrating mistakes.
Introducing Our Web-Based OLED Icon Generator
To address this need, we’ve developed a powerful web-based OLED icon generator that works across all devices. Whether you’re using a desktop computer, tablet, or smartphone, our tool provides an intuitive interface for creating beautiful icons for your OLED displays.
The tool features a responsive grid where you can toggle pixels on and off with simple clicks or taps. Additionally, it offers various editing tools like inversion, shifting, and rotation to help you perfect your designs. Once satisfied, you can instantly generate the Arduino-compatible code needed to display your creation.
Furthermore, our OLED icon generator supports multiple display sizes and configurations, making it versatile enough for virtually any project. Whether you’re working with a tiny 0.96-inch display or a larger 1.3-inch screen, our tool adapts to your needs.
17 Essential Techniques for Creating Stunning OLED Icons
Now, let’s explore the seventeen techniques that will transform the way you use an OLED icon generator for your projects:
1. Start with Simple Shapes
When beginning with an OLED icon generator, start with basic shapes like circles, squares, and triangles. These fundamental elements form the building blocks of more complex designs. Moreover, simple shapes render clearly even on small displays, ensuring visibility.
2. Embrace Negative Space
Negative space is particularly effective on OLED displays because of their perfect black levels. Consequently, designing icons that utilize both illuminated and dark areas creates striking visual contrast. Try creating icons where the meaning emerges from what isn’t lit rather than what is.
3. Optimize for Display Size
Different OLED displays have different resolutions. Therefore, always design with your specific display in mind. An 8×8 icon will appear tiny on a 128×64 display but might be perfect for a status indicator. Conversely, a detailed 32×32 icon might be ideal for a menu selection.
4. Create Consistent Icon Sets
When designing multiple icons for a project, maintain consistent styling across all graphics. As a result, your user interface will feel professional and cohesive. Use similar line weights, proportions, and design approaches throughout your set.
5. Test with Actual Hardware
The OLED icon generator provides a preview, but nothing beats seeing your designs on actual hardware. Consequently, test your icons on your target device throughout the design process. This practice helps identify issues with visibility, size, or clarity early.
6. Use Animation Frames
One powerful technique is creating sequential frames to produce simple animations. For instance, design 3-4 variations of an icon that can be displayed in sequence to create movement. A loading spinner or a blinking indicator adds polish to your projects.
7. Create Status Indicators
Design icon variations that indicate different states—for example, Wi-Fi icons showing different signal strengths or battery icons displaying various charge levels. These visual cues communicate information instantly without text.
8. Incorporate Text with Icons
Sometimes the most effective approach combines simple icons with text. Our OLED icon generator allows you to create custom text renderings that perfectly complement your graphical elements. This combination often communicates more clearly than either alone.
9. Use Dithering for Grayscale Effects
While most basic OLED displays are monochrome, you can simulate grayscale using dithering patterns. By alternating pixels in specific patterns, you create the illusion of different gray levels. This technique adds depth to your icons.
10. Design with Pixel Boundaries in Mind
Remember that OLED displays have physical pixels with defined boundaries. Therefore, diagonal lines can appear jagged. Use the OLED icon generator to preview how angled elements will render and adjust accordingly.
11. Create Recognizable Silhouettes
The most effective icons have distinctive silhouettes. When designing with an OLED icon generator, ensure your icons remain recognizable even when reduced to their outline. This approach ensures immediate recognition.
12. Implement Toggle States
Design pairs of icons that represent on/off or active/inactive states. For example, a speaker icon might have sound waves when on and none when muted. These paired designs create intuitive interfaces.
13. Use White Space Strategically
Don’t feel compelled to fill every pixel. Strategic use of empty space makes icons more legible and elegant. Remember, with OLEDs, unused pixels consume no power, so embrace minimalism where appropriate.
14. Optimize Memory Usage
When working with microcontrollers, memory efficiency matters. The OLED icon generator helps optimize your graphics for minimal memory footprint. Consider using smaller icon sizes or simplifying complex designs for memory-constrained projects.
15. Create Contextual Icons
Design icons that communicate their function within the context of your project. A generic “settings” icon works across many applications, but custom icons specifically designed for your project’s functions create a more intuitive experience.
16. Implement Scrolling for Larger Graphics
For detailed images that exceed your display size, implement scrolling techniques. The OLED icon generator can help create larger graphics that you can programmatically scroll across the screen for impressive visual effects.
17. Design with Scaling in Mind
Create icons that scale well to different sizes. This approach allows you to reuse designs across projects with different display resolutions. The OLED icon generator helps preview how your designs look at various scales.
Step-by-Step Guide to Using Our OLED Icon Generator
Now that you understand the key techniques, let’s walk through the process of creating an icon with our OLED icon generator:
Step 1: Select Your Canvas Size
Begin by choosing the dimensions that match your project requirements. Our tool supports various common OLED resolutions, including 8×8, 16×16, 32×32, and 128×64. Additionally, you can create custom-sized canvases for specialized applications.
Step 2: Design Your Icon
Use the interactive grid to toggle pixels on and off. Furthermore, take advantage of the drawing tools like lines, shapes, and fill functions to speed up the process. The real-time preview shows exactly how your icon will appear on an OLED display.
Step 3: Refine Your Design
Utilize the editing tools to perfect your creation. For instance, you can invert colors, shift the entire image, or mirror it horizontally or vertically. These refinements help achieve the perfect look with minimal effort.
Step 4: Generate the Code
Once satisfied, click the “Generate Code” button. The OLED icon generator automatically creates the Arduino-compatible code needed to display your icon. This code works with popular libraries like Adafruit_SSD1306 or U8g2.
Step 5: Copy and Implement
Finally, copy the generated code into your Arduino sketch. The OLED icon generator produces clean, optimized code that you can integrate immediately into your project. Just include the appropriate library, and your custom icon is ready to display.
Advanced Uses of OLED Icon Generator for Projects
Beyond basic icons, our OLED icon generator supports several advanced applications:
Creating User Interfaces
Design complete user interfaces with multiple icons, indicators, and text elements. By combining various graphics, you can create intuitive menu systems, status screens, and control panels for your projects.
Developing Games
The pixel-perfect control offered by our OLED icon generator makes it ideal for creating simple games for microcontroller platforms. Design game characters, obstacles, and environments with ease.
Data Visualization
Create custom graphics for representing data visually. From bar graphs to custom meters, the OLED icon generator helps you design unique ways to display information collected by your project’s sensors.
Educational Tools
Develop educational tools that use visual elements to explain concepts. The combination of text and custom graphics creates engaging learning experiences on even the smallest displays.
Troubleshooting Common OLED Display Issues
Even with a perfect icon from our OLED icon generator, you might encounter display issues. Here are solutions to common problems:
Problem: Icon Appears Inverted
Some OLED display libraries use different color conventions. If your icon appears inverted (black and white reversed), try using the invert function in the OLED icon generator before exporting the code.
Problem: Display Shows Nothing
Check your wiring and I2C/SPI address settings. Most OLED displays use specific addresses that must match in your code. Additionally, ensure you’ve initialized the display correctly before attempting to show your icon.
Problem: Icon Appears Distorted
Verify that your display resolution settings match your actual hardware. Moreover, check that you’re using the correct library commands to display the bitmap generated by the OLED icon generator.
Problem: Memory Errors
If your Arduino reports memory errors, your icon might be too large for the available RAM. Consider using smaller icons or storing them in program memory (PROGMEM) using the options in our OLED icon generator.
Future of OLED Displays in Maker Projects
The landscape of OLED technology continues to evolve rapidly. Consequently, our OLED icon generator evolves too, supporting new display types and features as they become available.
Color OLEDs are becoming more affordable and accessible to hobbyists. Therefore, we plan to expand our OLED icon generator to support color graphics in future updates. This advancement will open new possibilities for even more impressive project interfaces.
Additionally, flexible OLED technology is emerging in the maker space. These bendable displays create exciting opportunities for wearable projects and uniquely shaped devices. Our OLED icon generator will adapt to support these innovative display formats.
Conclusion: Elevate Your Projects with OLED Icon Generator
An OLED icon generator transforms the way you approach user interfaces in your microcontroller projects. By simplifying the creation of custom graphics, it unlocks new possibilities for interaction, information display, and visual appeal.
Our web-based OLED icon generator provides all the tools you need to design professional-quality icons for your projects. Furthermore, it generates optimized code that works seamlessly with popular microcontroller platforms and display libraries.
We encourage you to experiment with the seventeen techniques we’ve shared and discover your own creative approaches. Share your creations with the community and inspire others with what’s possible when you combine electronics with thoughtful visual design.
Remember, the most memorable projects combine technical excellence with visual appeal. With our OLED icon generator, you have everything needed to create microcontroller projects that not only function flawlessly but look amazing too.
Start creating your custom OLED graphics today and take your projects to the next level!
FAQs About OLED Icon Generators
Q: Do I need programming experience to use the OLED icon generator?
A: No programming experience is required to create icons. Our OLED icon generator produces ready-to-use code that you can simply copy into your Arduino sketch.
Q: What microcontrollers work with the generated code?
A: The code works with Arduino, ESP8266, ESP32, and most other microcontrollers that support standard OLED libraries like Adafruit_SSD1306 or U8g2.
Q: Can I create animated graphics with the OLED icon generator?
A: Yes! By creating multiple frames and displaying them sequentially in your code, you can create simple animations with graphics from our OLED icon generator.
Q: What OLED display sizes are supported?
A: Our OLED icon generator supports all common OLED display resolutions, including 128×64, 128×32, 64×48, and others. You can design for any size display.
Q: Is the OLED icon generator free to use?
A: Yes, our basic OLED icon generator is completely free to use. Advanced features may be available in a premium version for power users.
- Download the BlueBot Controller App here.
- Make sure you have a Bluetooth-enabled smartphone to pair with your project.
Downlaod Basic electronics e-Book Click Here
Visit : Home Page
Learn about other sensors, such as Arduino sensors.